Network call using Retrofit
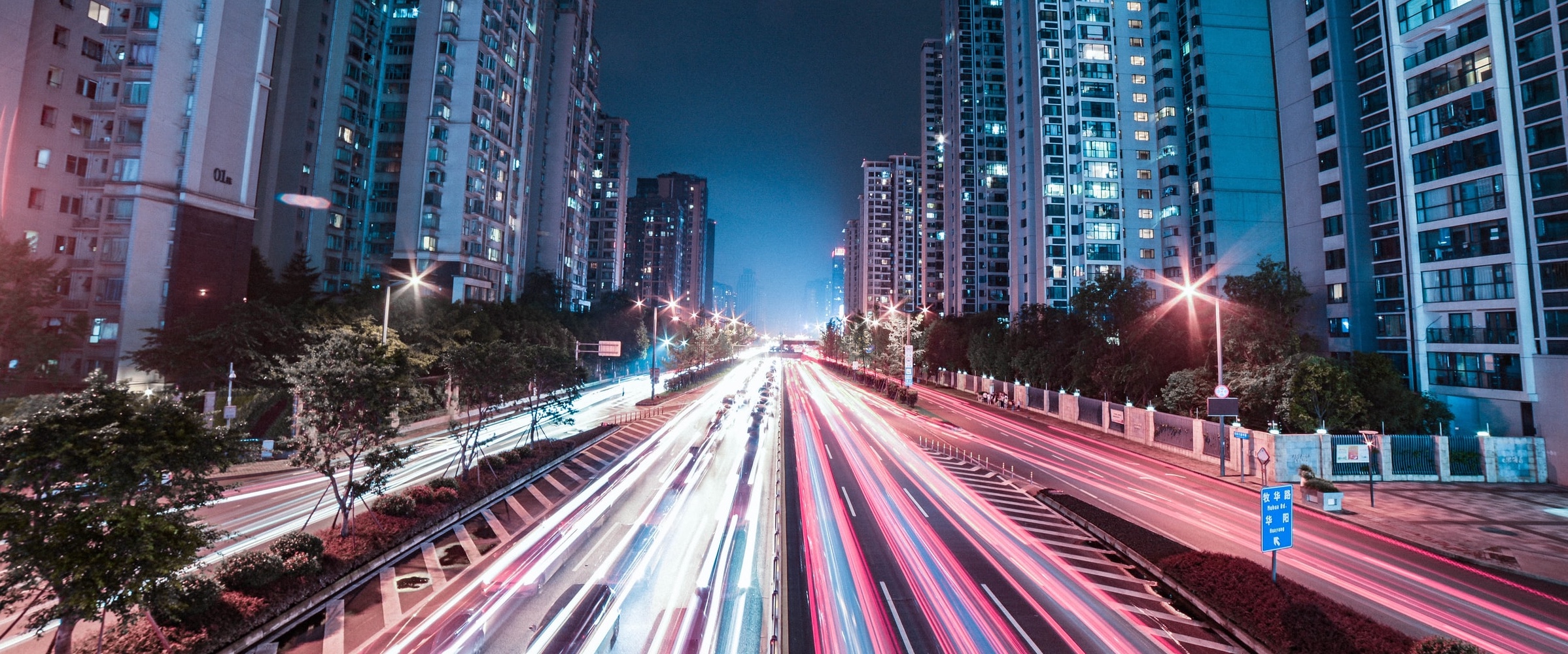
1. Setup
Add the libraries to the gradle dependencies and add the Internet permission on the Manifest.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<uses-permission android:name="android.permission.INTERNET" /> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// build.gradle (:app) | |
android { | |
//.. | |
compileOptions { | |
sourceCompatibility JavaVersion.VERSION_1_8 | |
targetCompatibility JavaVersion.VERSION_1_8 | |
} | |
} | |
dependencies { | |
//.. https://square.github.io/retrofit/ | |
implementation 'com.squareup.retrofit2:retrofit:2.9.0' | |
implementation 'com.squareup.retrofit2:converter-moshi:2.9.0' | |
} |
2. Using the library
Copy/paste the snippet below into the MainActivity.kt
file, ideally each class/interface would all have their own file,
but we'll keep it in the same one for now to keep it simple.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class MainActivity : AppCompatActivity() { | |
override fun onCreate(savedInstanceState: Bundle?) { | |
super.onCreate(savedInstanceState) | |
setContentView(R.layout.activity_main) | |
/* Creates an instance of the UserService using a simple Retrofit builder using Moshi | |
* as a JSON converter, this will append the endpoints set on the UserService interface | |
* (for example '/api', '/api?results=2') with the base URL set here, resulting on the | |
* full URL that will be called: 'https://randomuser.me/api' */ | |
val service = Retrofit.Builder() | |
.baseUrl("https://randomuser.me/") | |
.addConverterFactory(MoshiConverterFactory.create()) | |
.build() | |
.create(UserService::class.java) | |
/* Calls the endpoint set on getUsers (/api) from UserService using enqueue method | |
* that creates a new worker thread to make the HTTP call */ | |
service.getUsers().enqueue(object : Callback<UserResponse> { | |
/* The HTTP call failed. This method is run on the main thread */ | |
override fun onFailure(call: Call<UserResponse>, t: Throwable) { | |
Log.d("TAG_", "An error happened!") | |
t.printStackTrace() | |
} | |
/* The HTTP call was successful, we should still check status code and response body | |
* on a production app. This method is run on the main thread */ | |
override fun onResponse(call: Call<UserResponse>, response: Response<UserResponse>) { | |
/* This will print the response of the network call to the Logcat */ | |
Log.d("TAG_", response.body()) | |
} | |
}) | |
} | |
} | |
/* Kotlin data/model classes that map the JSON response, we could also add Moshi | |
* annotations to help the compiler with the mappings on a production app */ | |
data class UserResponse(val results: List<User>) | |
data class User(val email: String, val phone: String) | |
/* Retrofit service that maps the different endpoints on the API, you'd create one | |
* method per endpoint, and use the @Path, @Query and other annotations to customize | |
* these at runtime */ | |
interface UserService { | |
@GET("/api") | |
fun getUsers(): Call<UserResponse> | |
} |